|
Databases, Networks, and Graphics FAQ
|
|
- Useful links:
|
|
|
|
|
|
- FAQ:
NB This is an unofficial FAQ page and does not claim to contain perfectly correct information. If you detect any mistakes, please let me know the errors, and I will correct the mistakes as soon as possible. Thanks for your contribution! |
|
- How to start with Eclipse?
If you are using Eclipse, in order to build a project, go to "File > New > Java Project". Then, you will be asked to type a project name. Enter the same name as the example code, and click "Finish". You will find a project is being set up. Then, add a "class" in a source folder by using "new". After that, paste the code from our web site, don't forget to keep the same filename with the example class.
- How to read red, green, and blue values of a pixel?
First, you may want to call the "getRGB" function to read a pixel value in a form of a "Color" object.
Color inC = new Color(face.getRGB(i,j));
Then, we have to take four channels from the Color object as follows:
int inRed = inC.getRed();
int inGreen = inC.getGreen();
int inBlue = inC.getBlue();
int inAlpha = inC.getAlpha();
Once we have four individual colour coordinates, we can manipulate colour properties. Then, we set it back to a new image object by calling the "setColor" function as follows:
Color outC = new Color(inAver, inAver, inAver, inAlpha);
gbi.setColor(outC); gbi.drawLine(i,j,i,j);
- How to draw a Bezier curve by using a recursive split?
I cannot give you the whole answer of this coursework, though. I would help you to understand the Bezier curve. Bezier curves are one of the most common representations for free-form curves because we can make any curve with control in a sensible way. Good examples that use this Bezier curves are Adobe Illustrator and Postscript. The De Casteljau Algorithm is one of the easy ways to draw Bezier curves.
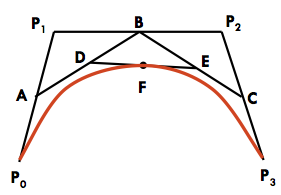
Here we have four initial points, called P0, P1, P2, and P3. Imagine that we can bisect each line between adjacent points such as P0-P1, P1-P2, and P2-P3. By connecting the bisected points, let's call them A, B, and C. We could bisect them again to obtain middle points D and E from the lines A-B and B-C. Finally, by connecting the middle points D and E, we have a line and bisect it to get a point F. This F is a centre point of this Bezier curve. Then, we could assume that we have two Bezier curves: a left curve that has four control points (P0, A, D, and F) and a right curve that has four control points (F, E, C, and P3). By keeping splitting the Bezier curve recursively, we could have multiple sub-Bezier curves to form a complete Bezier curve in total. In order to code this algorithm by yourself, you may want to use a recursive callback function.
-
Isn't there any ready-made object for 2D coordinates in Java?
2D coordinates can be represented and utilised by the"Point2D" class. If you want to use this, define "import" in the header part of your code as follows:
import java.awt.geom.Point2D;
Then, you can initialise a coordinate:
Point2D start = new Point2D.Double();
And you can call x or y coordinates as follows:
double sum = (start.x + start.y);
This would make your codes cleaner and more eligible.
- How can I draw a line on the Graphics2D objects?
You cannot draw a line on the Graphics2D objects; hence, you have to call a "GeneralPath" object intermediately:
java.awt.geom.GeneralPath lines = new java.awt.geom.GeneralPath();
Then, you can indicate two start and end points, A and B, of the path:
lines.moveTo(A.x, A.y);
lines.lineTo(B.x, B.y);
Finally, enter this path object into your Graphics2D objects:
g2d.draw(lines);
|
|
|
|
Hosted by Visual Computing Laboratory, School of Computing, KAIST.
|
 |
|